Discover the Magic of Trove
Add a dash of enchantment to your transaction data by sprinkling in merchant domain details, industry, and even location insights. 🌟
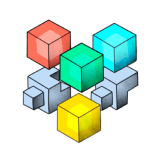
First, get your API KEY
Ready to Dive into APIs? Click below to create and copy over your API key. So you can paste in on this page to explore this interactive documentation page. Get My API KeysOverview
To enrich your bank or credit card transactions, Trove needs a few details in order to look up the merchant. To test your API request on this page, paste your Trove API key, then click on each of the input value area in the "REQUEST" section to edit and input your specific transaction related values. Afterwards, click on "Try It Out" and review your enriched transaction right on this page.
Please note that our API is specifically designed to optimize matching for US financial transactions in English. Transactions conducted in other languages or regions may result in less accurate matching.
Authentication
To authenticate your requests, first create an account on Trove and then create your personal API key.
X-API-KEY String required
This needs to be in your header with the key name being X-API-KEY and the value set to your personal API key.Enrich a merchant transaction
POST https://trove.headline.com/api/v1/transactions/enrich
Body Params
description String required
The original, unaltered bank or credit card transaction description string from the payment processor, including any asterisks and numbers.amount Float required
Transaction value in the original currency. Negative values indicate credit transactions.date String required
The transaction date in YYYY-MM-DD format.user_id String required
A unique identifier for the customer/user that performed this transaction such as user ID or hash. Please avoid sending PII such as email or the user's name.Request
Response
Responses
200 - Successful Operation
If the request is successful, you will receive the Response Body with the following attributes.Response Body
type String
The type of company the merchant is categorized as, such as "Public Company" or "Privately Held".name String
The name of the company.domain String
The domain of the company.founded String
The founding date of the company in format of YYYY-MM-DD.industry String
The industry of the company.size String
The approximate employee headcount of the company.hq_city String
The headquarter city of the company.hq_state String
The headquarter state of the company.hq_state_code String
The state of the company headquarter resides in. Formatted as USPS 2-letter code (only applicable to US companies).hq_country_code String
The 2-letter country code (ISO 3166-1) the company is based in.facebook_url String
The official Facebook page of the company.twitter_url String
The official Twitter (now X) page of the company.linkedin_url String
The official LinkedIn page of the company.categories Array
The business categories the company belongs to.id String
The user_id of the transaction that was originally sent to the request.400 - Bad Request
Bad request. Make sure all the required fields are present in the request body and are formatted properly.403- Forbidden request
Make sure the X-API-KEY header is present and valid.429 - Too many requests
Too many requests. The API rate limit has been reached for the provided API Key.Feedback for transaction enrichment
POST https://trove.headline.com/api/v1/transactions/feedback
Body Params
description String required
The original transaction description you submitted to /api/v1/transactions/enrich endpoint.domain String required
The correct domain you would like to associated with this transaction description.comment String
Your optional feedback on our enrichment data response.Request
Response
Responses
200 - Successful Operation
If the request is successful, you will receive below data back.Response Body
success String
Success response400 - Bad Request
Bad request. Make sure all the required fields are present in the request body and are formatted properly.403- Forbidden request
Make sure the X-API-KEY header is present and valid.Bulk transactions enrichment
POST https://trove.headline.com/api/v1/transactions/bulk
You can only submit 3 bulk transactions requests concurrently. And you can submit more once the previous requests are completed.
Body Params
description String required
The original, unaltered bank or credit card transaction description string from the payment processor, including any asterisks and numbers.amount Float required
Transaction value in the original currency. Negative values indicate credit transactions.date String required
The transaction date in YYYY-MM-DD format.user_id String required
A unique identifier for the customer/user that performed this transaction such as user ID or hash. Please avoid sending PII such as email or the user's name.Request
Response
Responses
201 - Created
If the request is successful, you will receive below data back.Response Body
requestId String
Request ID response400 - Bad Request
Bad request. Make sure all the required fields are present in the request body and are formatted properly.403 - Forbidden request
Make sure the X-API-KEY header is present and valid.429 - Too Many Requests
You reached the maximum number of pending bulk requests allowed.Requesting Bulk transaction result
GET https://trove.headline.com/api/v1/transactions/bulk/{requestId}